As a Drupal developer, when I worked on my first WordPress theme the first thing I noticed was the messy combination of HTML and PHP in the same file. In Drupal I use Twig to keep the two separate while maintaining communication between them, saving myself a big headache in the process! So the question is, is it possible to use Twig in WordPress?
The answer is yes—with a little help from Timber.
This step-by-step guide explains how you can use Timber and Twig together to code cleaner, faster, and smarter in WordPress. It’s ideal if you’re already familiar with PHP, Composer, Wordpress, and theme development.
Now let’s get stuck in!
What are Twig & Timber? A Quick Overview
Twig is a template engine that allows developers to have the logic in one file and the presentation layer in a different file. It also optimizes your code, which is ideal with a language as verbose as PHP.
Timber is a WordPress plugin that allows developers to include and leverage all the features of Twig. Tens of thousands of sites are using Timber, and it can be used on existing themes.
Step 1: Set Up Your WordPress Site & Install Timber
First, we’re going to set up a basic WordPress website and install Timber. Since version 2.0 it needs to be installed using Composer.
Timber can be installed either inside a theme folder or in the root WordPress folder. In this example, we’re going to install it in the root WordPress folder. Simply execute the following in the command line:
composer install timber/timber
Step 2: Create a New Custom Theme
To start using Timber, you’ll need to create a new custom theme. The structure of the theme we’ll use for this example is as follows:
- Timber-theme (folder)
- Views (folder)
- single.twig
- footer.php
- functions.php
- header.php
- index.php
- single.php
- style.css
- Views (folder)
If you need more information about the process, there is official documentation about theme creation in WordPress.
Step 3: Implement Timber
The files that we need to review more in detail are the functions.php, the single.php and the single.twig. The functions.php file includes the code that will load the Timber library in our theme:
<?php
// Load Composer dependencies.
require_once ABSPATH . '/vendor/autoload.php';
// Initialize Timber.
Timber\Timber::init();
We’ve only included this code in our example because we don’t have the theme or project set up to pull in Composer’s autoload file — otherwise it wouldn’t be needed. The code uses the variable ABSPATH because the package was installed in the root of the site as a project dependency. If it was installed as a theme dependency, this location would need to be changed. If the autoload file is not correctly loaded, then the next line of code (which is in charge of initializing the timber plugin) will fail and show an error.
The next file is the single.twig file. Let’s start this example with the classic “Hello world!”. We’ll update this file later.
<h1>Hello {{ who }}!</h1>
Assuming you’re familiar with Twig syntax, you’ll notice that the expression inside the brackets is a variable and we’re displaying its value next to the word “Hello”. But where does this information come from? Let’s check the single.php file.
<?php get_header(); ?>
<?php
$data = array( 'who' => 'world' );
Timber::render( 'single.twig', $data );
?>
<?php get_footer(); ?>
The fourth line generates an array. Notice that its key is ‘who’. The next line is where the magic happens. The Timber::render function takes two parameters — the name of the template to be rendered, and an array with data to be passed to the template.
Notice that the key of the array is available as a variable inside the Twig template and it printed the value that it contained. In general, the keys of the array which is passed as a parameter to the render function are converted to variables in the Twig template.
This short example shows how information flows from a regular WordPress PHP template to a Twig-Timber template. But a WordPress template has more contextual information which is part of the strength of its system. How can we leverage the same capabilities inside our Twig templates? By using context. Let’s update our example.
<?php get_header(); ?>
<?php
$context = Timber::context();
Timber::render( 'single.twig', $context );
?>
<?php get_footer(); ?>
<h1>{{ post.title }}</h1>
<div>{{ post.excerpt }}</div>
In this updated example, we’re not setting the data manually in an array. Instead, we’re using the Timber context which contains some of the information that’s already available in the template (like the post object). We’re sending that information to the template, making it possible to use those objects in Twig. With this change, information flows from the WordPress backend directly to the Twig template. Here’s how the updated example looks:
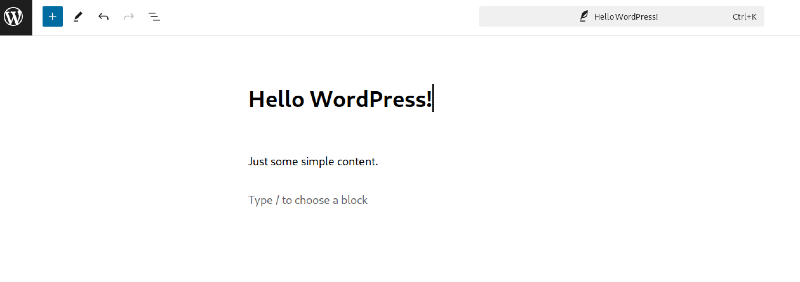
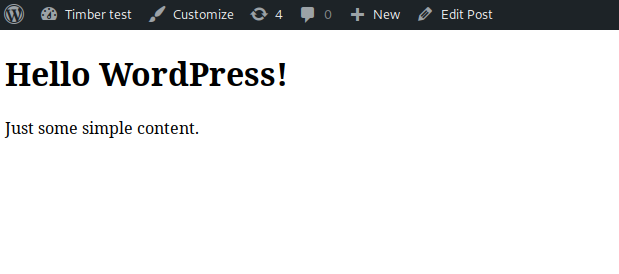
Additional Functionality and Benefits
This is just a simple example of how you can implement Timber in a project. But there are even more benefits to using Timber in your next WordPress website, including:
- Timber-Twig’s extensive functionality. Twig provides functions, filters, inheritance, and even more from Timber which integrates with native features from WordPress like shortcodes. You can learn more from the official Timber website and GitHub’s Timber documentation.
- Better separation of front-end and back-end. A front-end developer can work in the template structure in Twig at the same time as another developer works on the back-end logic.
- Improved security on the output. In a traditional WordPress template you have to use escaping functions to print the information. Twig automatically auto-escapes unicode and special characters.
- More familiarity with other template engines. There are other frameworks that use a similar handlebars syntax to Twig, such as Blade, meaning you’ll gain transferable knowledge.
Want to take your development skills to the next level? Get hands-on instruction from our Drupal and WordPress experts. Browse our training courses for teams and individuals.